Contact Synchronization
Get, create, and update contacts using Alloy's common model for CRMs.
Use Case Overview
Key Model(s) and Fields | Supported Applications |
---|---|
ContactsfirstName lastName account emailAddress remoteUpdatedAtMin remoteUpdatedAtMax | Salesforce CRM, HubSpot, MS Dynamics CRM, Zoho CRM |
Data Flow: Your App <-> CRM
This is a bi-directional integration. In this example, you can GET, POST, PUT/PATCH and DELETE target resources to help users maintain a clean database.
How to build this use case with Alloy's Unified API
1. Create a Connection
To get started, you'll need to first implement Alloy's Unified API and create a connection. A connection represents each time an end user links an app to Alloy's Unified API. See Creating a Connection in our Unified API Quickstart.
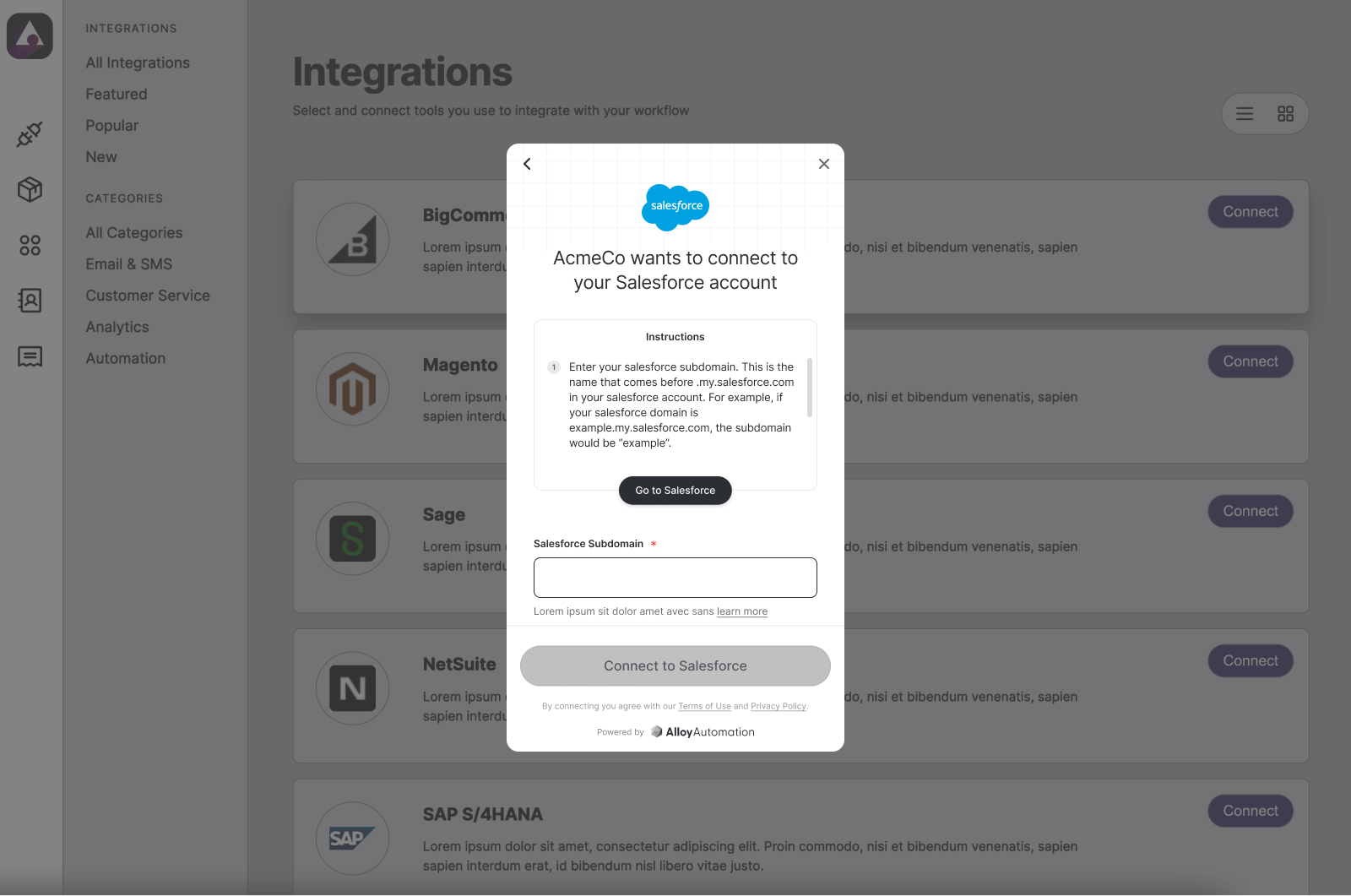
Your end-users authenticate integrations using the Alloy Modal.
If you’ve already done this, read on!
2. Sync historical contact data from a user's CRM to your application
The first step is to sync the existing contacts in your user's CRM to your application/database.
Call the [GET List Contacts]
endpoint to retrieve your desired list of contacts from the CRM. If you don’t want to sync every contact — for example, maybe you’re only interested in those created after a certain date — you can filter queries with parameters like remoteCreatedAtMin
, remoteCreatedAtMax
, remoteUpdatedAtMin
, and remoteUpdatedAtMax
.
curl --request GET \
--url 'https://embedded.runalloy.com/2024-03/one/crm/contacts?credentialId=CREDENTIAL_ID&remoteUpdatedAtMin=2022-11-19T00:00:00.000Z&remoteUpdatedAtMax=2023-11-20T00:00:00.000Z' \
--header 'Authorization: Bearer YOUR_API_KEY' \
--header 'accept: application/json'
Your response payload should look something like this:
{
"contacts": [
{
"remoteId": "0000000000000000000",
"firstName": "Gregg",
"lastName": "Mojica",
"account": null,
"addresses": [],
"emailAddresses": [],
"phoneNumbers": [],
"lastActivityAt": null,
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z",
"id": "b8f78a04-b00d-4192-ba4f-935a72ac6b4d"
},
{
"remoteId": "0000000000000000000",
"firstName": "Jonathan",
"lastName": "Lee",
"account": null,
"addresses": [
{
"country": "",
"street1": "",
"state": "",
"city": "",
"addressType": "mailing",
"postalCode": ""
}
],
"emailAddresses": [],
"phoneNumbers": [],
"lastActivityAt": null,
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z",
"id": "c3d2c3po-l00r-8892-re7t-734f01fh90kl"
},
{
"remoteId": "0000000000000000000",
"firstName": "Dave",
"lastName": "Smith",
"account": null,
"addresses": [],
"emailAddresses": [],
"phoneNumbers": [],
"lastActivityAt": null,
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z",
"id": "74g338fc-192h-2738-7384-23e44hgf9f87"
},
{
"remoteId": "0000000000000000000",
"firstName": "Viral",
"lastName": "Ruparel",
"account": null,
"addresses": [],
"emailAddresses": [],
"phoneNumbers": [],
"lastActivityAt": null,
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z",
"id": "3827501c-87g7-3g39-72fc-hfd775dhc716"
}
]
}
As you can see, your response payload will match the structure of Alloy’s common model for Contacts, rather than the structure of the source application (Salesforce, HubSpot, Dynamics or Zoho).
From here, you can write the app logic to sync the contacts to your application’s database.
3. Writing contacts to your user's CRM
Use the [POST Create Contact]
endpoint to write new contacts that are generated in your application to your user's CRM. You can also choose and filter by a unique identifier to avoid the creation of duplicate records — some of which include:
emailAddress
id
createTimestamp
Here's what the request looks like:
curl --request POST \
--url 'https://embedded.runalloy.com/2023-06/one/crm/contacts?credentialId=CREDENTIAL_ID' \
--header 'Authorization: Bearer YOUR_API_KEY' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"lastName": "Lee",
"firstName": "Jon",
"account": null,
"emailAddresses": [
{
"emailAddress": "[email protected]",
"emailAddressType": "Main"
}
],
"phoneNumbers": [
{
"phoneNumberType": "Cell",
"phoneNumber": "(111)222-3333"
}
]
}
'
And here's the example response:
{
"contact": {
"remoteId": "003Hs00004kyNdFIAU",
"firstName": "Jon",
"lastName": "Lee",
"account": null,
"addresses": [],
"emailAddresses": [],
"phoneNumbers": [
{
"phoneNumberType": "Phone",
"phoneNumber": "(111)222-3333"
}
],
"lastActivityAt": null,
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z",
"id": "6a47a18f-3506-43f4-806d-08d567bdc50b"
}
}
4. Updating existing contacts
To update existing Contacts, use the PUT Update Contacts
endpoint to generate a request that looks like this:
curl --request PUT \
--url 'https://embedded.runalloy.com/2023-06/one/crm/contacts/12?credentialId=CREDENTIAL_ID' \
--header 'Authorization: Bearer YOUR_API_KEY' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"firstName": "Joe",
"lastName": "Smith",
"emailAddresses": [
{
"emailAddressType": "Main",
"emailAddress": "[email protected]"
}
]
}
'
In both cases, the Contacts you write will be structured using Alloy’s common model.
Ensure that users have accurate contact data
By ensuring that contact data flows smoothly between your product and a user’s CRM, you can ensure that they’re always working with the most up-to-date information and creating the personalized experiences their customers expect.
Updated about 1 month ago