Accounts Receivable Automation
Automate the accounts receivable workflow for users by integrating with their ERP and accounting platforms.
Use Case Overview
Key Model(s) and Fields | Supported Applications |
---|---|
InvoicescustomerId trackingCategories totalAmount lineItems Customers id customer taxNumber email addresses Payments totalAmount vendorId trackingCategoryIds | NetSuite, QuickBooks, Microsoft Dynamics Business Central |
Data Flow: Your App <-> ERP/Accounting Platform
This is a bi-directional integration. In this example, you can GET and POST target resources to help users automate accounts receivable.
How to build this use case with Alloy's Unified API
1. Create a Connection
To get started, you'll need to first implement Alloy's Unified API and create a connection. A connection represents each time an end user links an app to Alloy's Unified API. See Creating a Connection in our Unified API Quickstart.
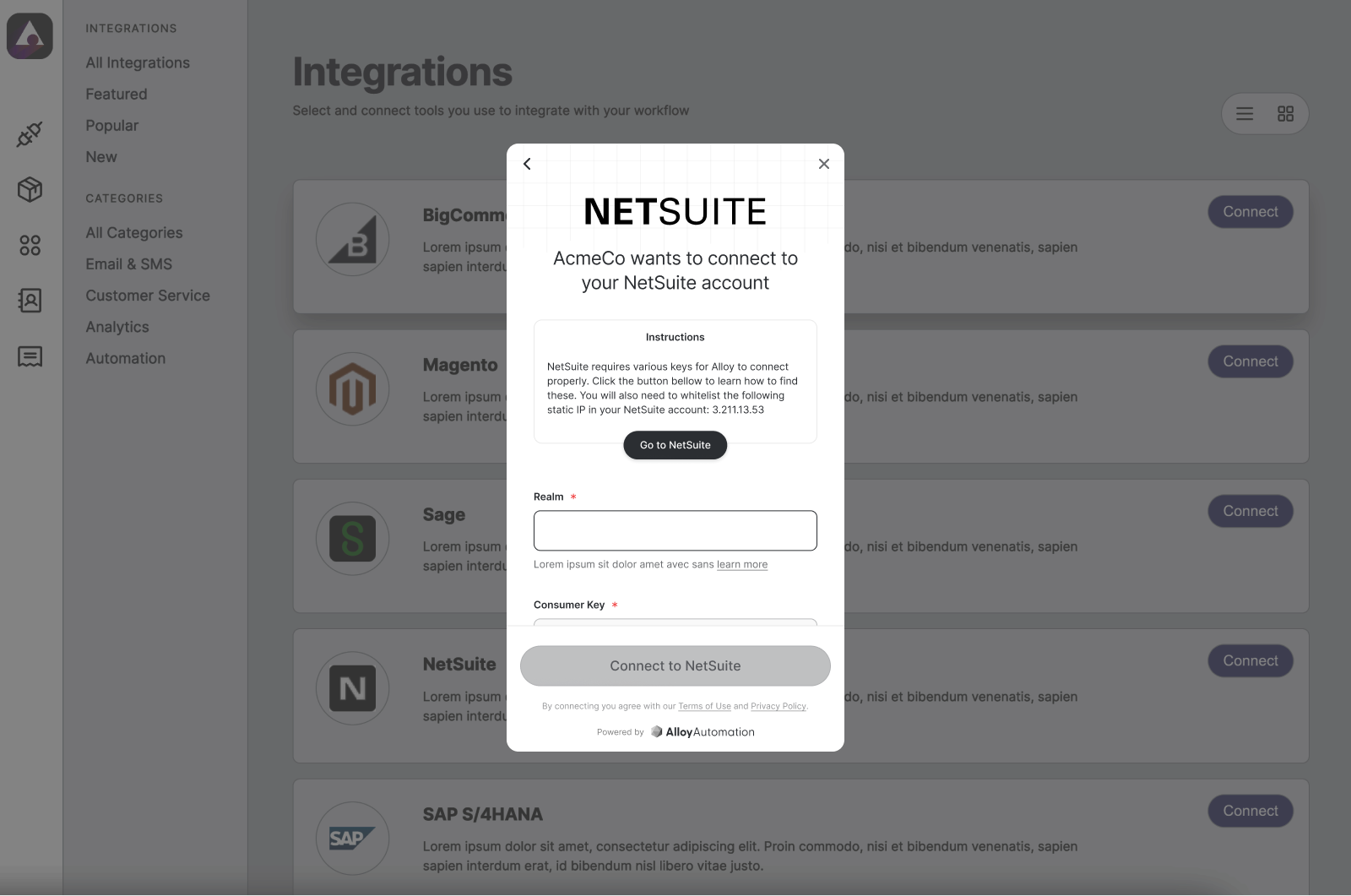
Your end-users authenticate integrations using the Alloy Modal.
If you’ve already done this, read on!
2. Sync customers between applications
Make sure that your application and your user’s Accounting platform have a matching database of customers. You can do this by using the GET List Customers
and POST Create Customer
endpoints, which will allow you to get all customers from your user’s Accounting platform and write customers to those platforms, respectively.
Your request to the GET List Customers
endpoint should look like this:
curl --request GET \
--url 'https://embedded.runalloy.com/2024-03/one/accounting/customers?credentialId=CREDENTIAL_ID' \
--header 'Authorization: bearer YOUR_API_KEY' \
--header 'accept: application/json'
Which will return a response payload structured like this:
{
"customers": [
{
"id": "00000000-0000-0000-0000-00000000",
"remoteId": "96",
"customerName": "Rushin123 Shah",
"email": null,
"taxNumber": null,
"customerStatus": "ACTIVE",
"currency": "USD",
"companyId": null,
"addresses": [],
"phoneNumbers": [],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
},
{
"id": "00000001-0000-0000-0000-00000001",
"remoteId": "97",
"customerName": "Emily Johnson",
"email": "[email protected]",
"taxNumber": "EJ1234567",
"customerStatus": "ACTIVE",
"currency": "USD",
"companyId": "EJCO",
"addresses": [
{
"zipCode": "10001",
"street1": "123 Broadway",
"state": "NY",
"city": "New York",
"addressType": "Billing",
"countrySubdivision": "NY"
}
],
"phoneNumbers": [
{
"phoneNumberType": "Primary",
"phoneNumber": "(212) 555-1234"
}
],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
},
{
"id": "00000002-0000-0000-0000-00000002",
"remoteId": "98",
"customerName": "John Doe",
"email": "[email protected]",
"taxNumber": "JD2345678",
"customerStatus": "PENDING",
"currency": "USD",
"companyId": "JDCO",
"addresses": [
{
"zipCode": "90001",
"street1": "456 Sunset Blvd",
"state": "CA",
"city": "Los Angeles",
"addressType": "Shipping",
"countrySubdivision": "CA"
}
],
"phoneNumbers": [
{
"phoneNumberType": "Mobile",
"phoneNumber": "(323) 555-6789"
}
],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
}
]
}
To create new customers in your user’s Accounting platform, make a request to the POST Create Customer
endpoint. The request should look something like this:
curl --request POST \
--url https://embedded.runalloy.com/2023-12/one/accounting/customers?credentialId=CREDENTIAL_ID \
--header 'Authorization: bearer YOUR_API_KEY' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"addresses": {
"addressType": "Billing",
"street1": "213 Example Ave",
"city": "New York",
"state": "NY",
"country": "US",
"zipCode": "10012"
},
"phoneNumbers": {
"phoneNumberType": "PRIMARY",
"phoneNumber": "12345678901"
},
"customerName": "Hooli",
"customerStatus": "ACTIVE",
"currency": "USD"
}
'
3. Create invoices in your user’s Accounting platform
When a user creates a new invoice in your application, you’ll need to create a corresponding record in their Accounting platform. You can do this with Alloy Unified API’s POST Create Invoice
endpoint.
See the request below:
curl --request POST \
--url https://embedded.runalloy.com/2023-12/one/accounting/invoices?credentialId=CREDENTIAL_ID \
--header 'Authorization: bearer YOUR_API_KEY' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"customerId": "{customerId}",
"lineItems": [
{
"description": "November Services",
"totalAmount": "2373.45"
}
],
"invoiceNumber": "7452",
"issueDate": "2023-12-10",
"dueDate": "2023-12-27",
"currency": "USD",
"balance": 2373.45
}
'
As you can see, the Invoice model in Alloy’s Unified API includes lineItems
, amount
, balance
and more — customerId
will be used to associate the invoice with a user’s customer. See our API reference for a full list of properties.
3. Get payment data from a user’s Accounting platform
To close the loop and present current customer balance information in your application, you’ll need to get payments made against invoices and reflect the appropriate invoice status (open, paid etc.) in your application.
To do this, make a call to the GET List Payments
endpoint. Your request should look like this:
curl --request GET \
--url https://embedded.runalloy.com/2023-12/one/accounting/payments?credentialId=CREDENTIAL_ID \
--header 'Authorization: bearer YOUR_API_KEY' \
--header 'accept: application/json'
Which will return a response that looks like this:
{
"payments": [
{
"id": "00000000-0000-0000-0000-00000000",
"remoteId": "33",
"transactionDate": "2021-04-30",
"customerId": "a9d9e59e-d553-4d91-b62b-ee7bf70772d8",
"vendorId": null,
"accountId": null,
"currency": "USD",
"exchangeRate": 1,
"companyId": null,
"totalAmount": null,
"trackingCategoryIds": [],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
},
{
"id": "00000001-0000-0000-0000-00000001",
"remoteId": "34",
"transactionDate": "2021-05-15",
"customerId": "b0d0f60f-e654-4c2d-9c3c-fa7bf81873e9",
"vendorId": null,
"accountId": "001",
"currency": "USD",
"exchangeRate": 1,
"companyId": "COMP01",
"totalAmount": 500,
"trackingCategoryIds": ["001", "002"],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
},
{
"id": "00000002-0000-0000-0000-00000002",
"remoteId": "35",
"transactionDate": "2021-06-01",
"customerId": "c1e1g71g-f765-4f8c-9d4d-ga8cf92984fa",
"vendorId": null,
"accountId": "002",
"currency": "USD",
"exchangeRate": 1,
"companyId": "COMP02",
"totalAmount": 750,
"trackingCategoryIds": ["003"],
"remoteCreatedAt": "2023-11-19T17:00:17.000Z",
"remoteUpdatedAt": "2023-11-19T17:00:17.000Z",
"remoteDeleted": false,
"createdAt": "2024-02-22T11:17:53.264Z",
"updatedAt": "2024-02-22T13:54:03.445Z"
}
]
}
From here, you can list any invoices with a non-zero balance as “Open” in your application, while invoices with no outstanding balance can be marked as “Paid.” Your users can then reconcile payments with invoices in your application and remind customers with open invoices to pay.
Help users automate accounts receivable
Automate the accounts receivable workflow to help users avoid billing disputes, get paid faster, and expand your product’s utility.
Updated 4 months ago